Handling comma-separated values (CSV) is a common requirement in database management, especially when working with data that needs to be parsed into multiple rows for easier processing, analysis, or integration. For example, you may encounter scenarios where a single column in a database contains delimited values , and you need to break these values into individual rows for further processing.
In SQL Server, we can efficiently convert such comma-separated values into multiple rows using a Common Table Expression (CTE).
Why Do We Need This:-
Normalization: De normalized data (comma-separated values in a single column) can be harder to manage and query. Splitting them into rows aligns with database normalization principles.
Simpler Queries: Queries and operations (e.g., aggregations, filtering) become more straightforward when working with separate rows rather than parsing string data repeatedly.
Scalability: Converting CSV data into rows allows you to integrate and analyze data more effectively, especially in large-scale datasets.
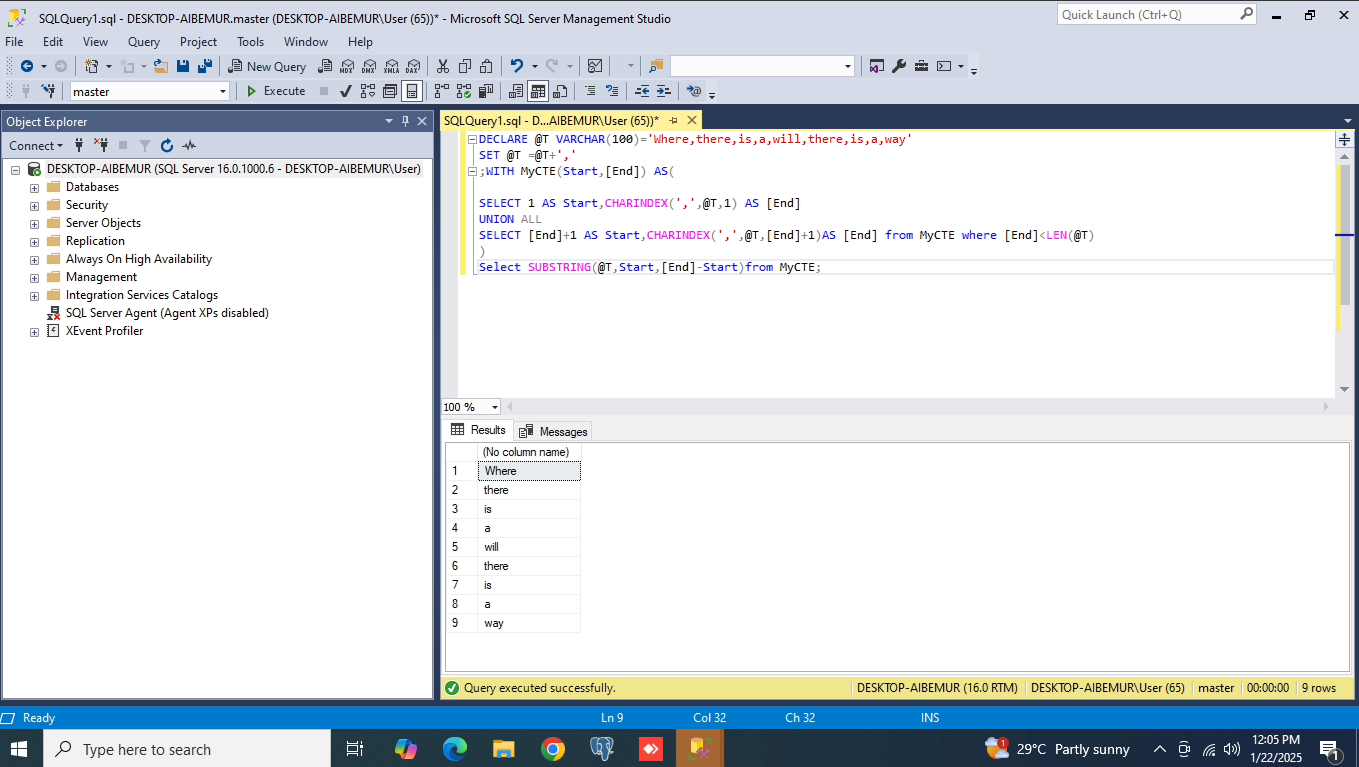
DECLARE @T VARCHAR(100) = ‘Where,there,is,a,will,there,is,a,way’;— Append a comma at the end to simplify parsingSET @T = @T + ‘,’;;WITH MyCTE(Start, [End]) AS (— Anchor Member: Find the position of the first commaSELECT1 AS Start,CHARINDEX(‘,’, @T, 1) AS [End]UNION ALL— Recursive Member: Continue finding commas after the previous oneSELECT[End] + 1 AS Start,CHARINDEX(‘,’, @T, [End] + 1) AS [End]FROM MyCTEWHERE [End] < LEN(@T) — Stop when the last comma is reached)— Extract substrings based on the calculated Start and End positionsSELECTSUBSTRING(@T, Start, [End] – Start) AS ValueFROM MyCTEWHERE [End] > 0; — Ensure valid positions